Overview
When you expose an Object Info (orange pin) to the group input, you will receive the option to choose between multiple geometry types within unreal.
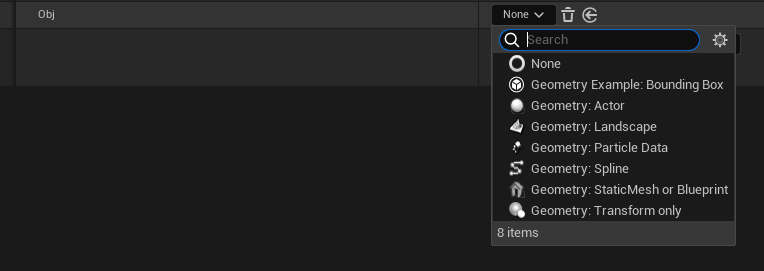
Static Mesh asset or Blueprint
This geometry type will allow you to select an asset, all the meshes inside this asset will be exported to blender. If you keep the object as instances (not realized) within your node group, they will be replaced by instances of the selected asset upon map save.
Transform override: allows you to make modifications to the transform of the exported mesh, such as rotating or scaling.
LOD: Which lod of the static mesh to export
Pay attention to the input “As Instance” of the Object Info node if you want to enable instancing
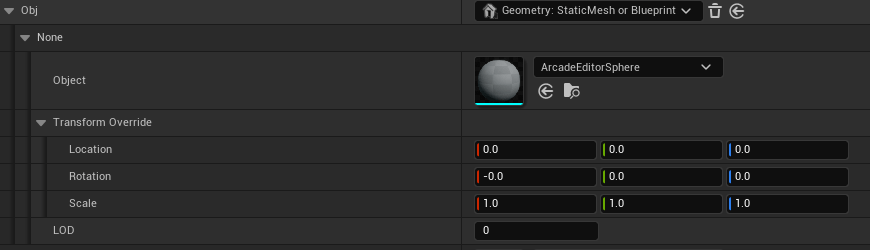
Transform Only
Equivalent to the “Empty” object in blender, it will only export transform information to your node setup, generally used as a pivot or as a target.
Draw As: how you want to visualize the transform, such as a cube or a single point.

Actor
This will export the selected actor to blender, including its mesh and transform information.
Use this input if you need the location of the actor to be available to your geometry nodes setup.
Use this input if you need the location of the actor to be available to your geometry nodes setup.

Landscape
This will export the landscape geometry to blender. Use this input if you need to place assets on top of the landscape.

Spline
Creates a spline curve to be edited in Unreal. Exposed params with a curve in its modifier defaults will be defaulted to this geometry type.
Resolution: How many points per segment the curve will have in Blender.

Collection
When a collection pin is exposed, it will become an array of geometry types, so you can mix and match Assets, Actors, etc.
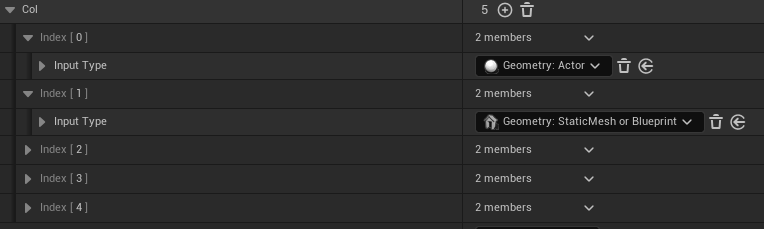
Expanding Geometry Types
Your project may create new geometry types (C++) if you need extra functionality.
This is an advanced feature
// .h
// Example Geometry type that exports the bounding box of the selected actor
// Must be paired with a file in ThirdParty/Extensions folder
UCLASS(meta=(DisplayName="Geometry Example: Bounding Box"))
class ALTERMESHEXAMPLESEDITOR_API UAlterMeshExamplesGeometryBoundingBox : public UAlterMeshGeometryBase
{
GENERATED_BODY()
public:
UPROPERTY(EditAnywhere, BlueprintReadWrite)
AActor* Actor;
virtual bool ShouldExport() override { return !!Actor; }
virtual void Export(FAlterMeshExport& Exporter) override;
};
// .cpp
void UAlterMeshExamplesGeometryBoundingBox::Export(FAlterMeshExport& Exporter)
{
Super::Export(Exporter);
FVector Origin, Extent;
Actor->GetActorBounds(false, Origin, Extent);
FBox Bounds = FBox::BuildAABB(FVector::ZeroVector, Extent);
// Create a cube from bounds
TArray Vertices;
TArray Indices;
UAlterMeshExamplesLibrary::MakeCube(Bounds, Vertices, Indices);
// Convert scene
for (FVector& Vertex : Vertices)
{
Vertex = Exporter.ToBlenderMatrix.TransformPosition(Vertex);
}
Exporter.WriteArray(Vertices);
Exporter.WriteArray(Indices);
}
The exporting code must be paired with a python file with the same name in the AlterMesh/Source/ThirdParty/ folder that will be used to import your geometry in Blender:
import bpy
from library import Reader
import numpy as np
def import_obj(object_name):
locations = Reader(np.float32).as_list(3)
indices = Reader(np.int32).as_array(3)
#create a mesh
mesh = bpy.data.meshes.new("NewMesh")
obj = bpy.data.objects.new(mesh.name, mesh)
mesh.from_pydata(locations, [], indices)
return obj
def used_for_object(obj):
False
def get_defaults(obj):
pass